UPDATE: Please use the latest jQuery plugin: jQuery YouTube Popup Player Plugin
YouTube provides a very neat customizable player to embed on your web pages, but not always you want most of your page area consumed by YouTube Video windows. So for that reason I have created an easy to use YouTube Popup window which can be embedded dynamically into the page just by clicking a link.
Using the popup div tag concept from my previous post, I have modified it to create dynamic div tags using JavaScript which makes it easier to embed multiple videos on the page without creating static div containers for the videos. To embed a video you just need to create any kind of image or text link which calls the JavaScriptopenYouTube() function on mouse click to create dynamic popup window. The link should appear something like this:
<a href="#" onclick="openYouTube('video_id')">Video Title</a>
Where video_id can be extracted from YouTube URL’s as shown in red below:
http://www.youtube.com/watch?v=_AJ0SkbPxAk
http://www.youtube.com/watch?v=EuAVgWJ28Hw&feature=related Video Id consists of all the characters between “v=” and “&” (if any).
http://www.youtube.com/watch?v=_AJ0SkbPxAk
http://www.youtube.com/watch?v=EuAVgWJ28Hw&feature=related Video Id consists of all the characters between “v=” and “&” (if any).
Advantages of using this popup YouTube player:
- Zero coding required!!!
- Piece of cake to embed YouTube popup windows by just calling the openYouTube() function from any link/button.
- Easily customizable YouTube parameters in the JavaScript function.
- No static Div tags required for each YouTube link.
This is how the Popup YouTube player looks like: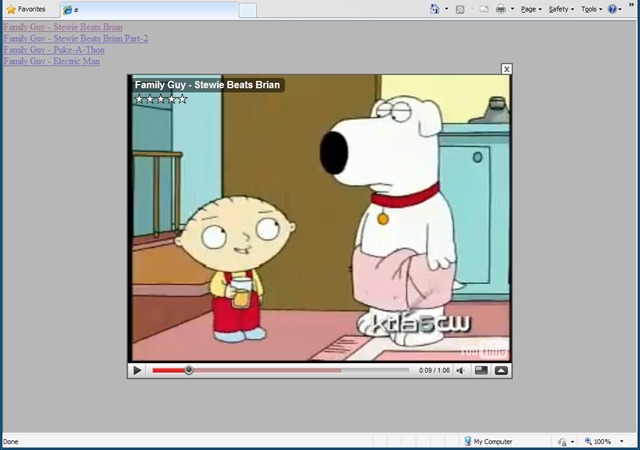
The openYouTube() function contains customizable YouTube parameters like video width & height, full screen control, auto play control and high definition playback control. You can modify this function and pass all these YouTube parameters as function parameters in openYouTube() which will give you individual control over all the videos on a single page.
UPDATE: Please use the latest jQuery plugin: jQuery YouTube Popup Player Plugin
Popup YouTube Player JavaScript code: <script language="javascript"> function openYouTube(id){
//YouTube Player Parameters
var width = 640;
var height = 505;
var FullScreen = "yes";
var AutoPlay = "yes";
var HighDef = "yes";
//Calculate Page width and height
var pageWidth = window.innerWidth;
var pageHeight = window.innerHeight;
if (typeof pageWidth != "number"){
if (document.compatMode == "CSS1Compat"){
pageWidth = document.documentElement.clientWidth;
pageHeight = document.documentElement.clientHeight;
} else {
pageWidth = document.body.clientWidth;
pageHeight = document.body.clientHeight;
}
}
// Make Background visible...
var divbg = document.getElementById('bg');
divbg.style.visibility = "visible";
//Create dynamic Div container for YouTube Popup Div
var divobj = document.createElement('div');
divobj.setAttribute('id',id); // Set id to YouTube id
divobj.className = "popup";
divobj.style.visibility = "visible";
var divWidth = width + 4;
var divHeight = height + 20;
divobj.style.width = divWidth + "px";
divobj.style.height = divHeight + "px";
var divLeft = (pageWidth - divWidth) / 2;
var divTop = (pageHeight - divHeight) / 2 - 10;
//Set Left and top coordinates for the div tag
divobj.style.left = divLeft + "px";
divobj.style.top = divTop + "px";
//Create dynamic Close Button Div
var closebutton = document.createElement('div');
closebutton.style.visibility = "visible";
closebutton.innerHTML = "<span onclick=\"closeYouTube('" + id +"')\" class=\"close_button\">X</span>";
//Add Close Button Div to YouTube Popup Div container
divobj.appendChild(closebutton);
//Create dynamic YouTube Div
var ytobj = document.createElement('div');
ytobj.setAttribute('id', "yt" + id);
ytobj.className = "ytcontainer";
ytobj.style.width = width + "px";
ytobj.style.height = height + "px";
if (FullScreen == "yes") FullScreen="&fs=1"; else FullScreen="&fs=0";
if (AutoPlay == "yes") AutoPlay="&autoplay=1"; else AutoPlay="&autoplay=0";
if (HighDef == "yes") HighDef="&hd=1"; else HighDef="&hd=0";
var URL = "http://www.youtube.com/v/" + id + "&hl=en&rel=0&showsearch=0" + FullScreen + AutoPlay + HighDef;
var YouTube = "<object width=\"" + width + "\" height=\"" + height + "\">";
YouTube += "<param name=\"movie\" value=\"" + URL + "\"></param>";
YouTube += "<param name=\"allowFullScreen\" value=\"true\"></param><param name=\"allowscriptaccess\" value=\"always\"></param>";
YouTube += "<embed src=\"" + URL + "\" type=\"application/x-shockwave-flash\" ";
YouTube += "allowscriptaccess=\"always\" allowfullscreen=\"true\" width=\""+ width + "\" height=\"" + height + "\"></embed></object>";
ytobj.innerHTML = YouTube;
//Add YouTube Div to YouTube Popup Div container
divobj.appendChild(ytobj);
//Add YouTube Popup Div container to HTML BODY
document.body.appendChild(divobj);
}
function closeYouTube(id){
var divbg = document.getElementById('bg');
divbg.style.visibility = "hidden";
var divobj = document.getElementById(id);
var ytobj = document.getElementById("yt" + id);
divobj.removeChild(ytobj); //remove YouTube Div
document.body.removeChild(divobj); // remove Popup Div
}
</script>
Popup YouTube Div CSS code: <style type="text/css">
<!--
.popup {
position: absolute;
z-index: 2;
}
.popup_bg {
position: absolute; visibility: hidden;
height: 100%; width: 100%;
filter: alpha(opacity=70); /* for IE */
opacity: 0.7; /* CSS3 standard */
left: 0px; top: 0px;
background-color: #999;
z-index: 1;
}
.ytcontainer {
border: 2px solid #666;
clear: both;
}
.close_button {
font-family: Verdana,Geneva , sans-serif;
font-size: small; font-weight: bold;
color: #666; text-decoration: none;
display: block; float: right;
background-color: #FFF;
z-index: 3; cursor: default;
border: 2px solid #666;
margin-bottom: -2px;
padding: 0px 3px 0px 3px;
}
body { margin: 0px; }
-->
</style>
<!--
.popup {
position: absolute;
z-index: 2;
}
.popup_bg {
position: absolute; visibility: hidden;
height: 100%; width: 100%;
filter: alpha(opacity=70); /* for IE */
opacity: 0.7; /* CSS3 standard */
left: 0px; top: 0px;
background-color: #999;
z-index: 1;
}
.ytcontainer {
border: 2px solid #666;
clear: both;
}
.close_button {
font-family: Verdana,
font-size: small; font-weight: bold;
color: #666; text-decoration: none;
display: block; float: right;
background-color: #FFF;
z-index: 3; cursor: default;
border: 2px solid #666;
margin-bottom: -2px;
padding: 0px 3px 0px 3px;
}
body { margin: 0px; }
-->
</style>
HTML code to embed YouTube Popup windows: <a href="#" onclick="openYouTube('_AJ0SkbPxAk')">Stewie Beats Brian</a><br />
<a href="#" onclick="openYouTube('EuAVgWJ28Hw')">Stewie Beats Brian Part-2</a><br/>
<a href="#" onclick="openYouTube('aRn5-LQCg2s')">Family Guy - Puke-A-Thon</a><br/>
<a href="#" onclick="openYouTube('5IQ9mpFZz9c')">Family Guy - Electric Man</a><br/>
<div id="bg" class="popup_bg"></div>
<a href="#" onclick="openYouTube('EuAVgWJ28Hw')">Stewie Beats Brian Part-2</a><br/>
<a href="#" onclick="openYouTube('aRn5-LQCg2s')">Family Guy - Puke-A-Thon</a><br/>
<a href="#" onclick="openYouTube('5IQ9mpFZz9c')">Family Guy - Electric Man</a><br/>
<div id="bg" class="popup_bg"></div>
You can put the CSS and JavaScript code in separate .CSS and .JS files to keep your HTML code neat and clean.